What happens when you attempt to compile and run the following code?
#include <iostream>
using namespace std;
class BaseC
{
public:
int *ptr;
BaseC() { ptr = new int(10);}
BaseC(int i) { ptr = new int(i); }
~BaseC() { delete ptr; }
};
void fun(BaseC x);
int main()
{
BaseC *o = new BaseC(5);
fun(*o);
}
void fun(BaseC x) {
cout << "Hello:"<<*x.ptr;
}
A. It prints: Hello:5
B. It prints: Hello:50
C. Compilation error
D. It prints: Hello:10
正解:A
質問 2:
What happens when you attempt to compile and run the following code?
#include <iostream>
#include <string>
using namespace std;
class A {
public:
A() { cout << "A no parameters";}
A(string s) { cout << "A string parameter";}
A(A &a) { cout << "A object A parameter";}
};
class B : public A {
public:
B() { cout << "B no parameters";}
B(string s) { cout << "B string parameter";}
B(int s) { cout << "B int parameter";}
};
int main () {
A a2("Test");
B b1(10);
B b2(b1);
return 0;
}
A. It prints: A no parametersA no parametersB string parameter
B. It prints: A string parameterA no parametersB int parameterA object A parameter
C. It prints: A no parametersA no parameters
D. It prints: A no parametersB string parameter
正解:B
質問 3:
What happens when you attempt to compile and run the following code?
#include <iostream>
#include <string>
using namespace std;
const int size = 3;
class A {
public:
string name;
A() { name = "Bob";}
A(string s) { name = s;}
A(A &a) { name = a.name;}
};
class B : public A {
public:
int *tab;
B() { tab = new int[size]; for (int i=0; i<size; i++) tab[i]=1;}
B(string s) : A(s) { tab = new int[size]; for (int i=0; i<size; i++) tab[i]=1;}
~B() { delete tab; }
void Print() {
for (int i=0; i<size; i++) cout << tab[i];
cout << name;
}
};
int main () {
B b1("Alan");
B b2;
b1.tab[0]=0;
b1.Print(); b2.Print();
return 0;
}
A. It prints: Alan
B. It prints: 111
C. It prints: 0
D. It prints: 011Alan111Bob
正解:D
質問 4:
What happens when you attempt to compile and run the following code?
#include <iostream>
using namespace std;
class Test {
float i,j;
};
class Add {
public:
int x,y;
Add (int a=3, int b=3) { x=a; y=b; }
int result() { return x+y;}
};
int main () {
Test test;
Add * padd;
padd = &test;
cout << padd?>result();
return 0;
}
A. It prints: 9
B. Compilation error
C. It prints: 6
D. It prints: 33
正解:B
質問 5:
How could you pass arguments to functions?
A. by value
B. by pointer
C. by void
D. by reference
正解:A,B,D
質問 6:
What is the expected result of the following program?
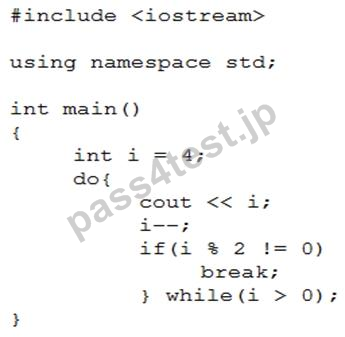
A. It prints: 420
B. It prints: 42
C. It prints: 4
D. The program enters an infinite loop
正解:B
質問 7:
What will happen if the memory cannot be allocated?
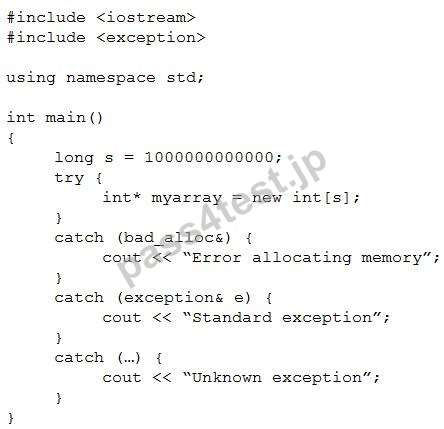
A. The program will print: Unknown exception
B. The program will cause a compilation error
C. The program will print: Error allocating memory
D. The program will print: Standard exception
正解:C
質問 8:
Which code, inserted at line 19, generates the output "23"?
#include <iostream>
#include <string>
using namespace std;
class A {
int x;
protected:
int y;
public:
int z;
A() { x=1; y=2; z=3; }
};
class B : public A {
string z;
public:
int y;
void set() { y = 4; z = "John"; }
void Print() {
//insert code here
}
};
int main () {
B b;
b.set();
b.Print();
return 0;
}
A. cout << B::y << B::z;
B. cout << y << z;
C. cout << A::y << A::z;
D. cout << y << A::z;
正解:C
Serizawa -
本格的なCPA-21-02問題も掲載されてるし、索引も充実!